Table of Contents
Introduction
OpenAI API Integration: I approached ChatGPT with a request to provide me with the necessary code for integrating an OpenAPI into my WordPress website using the API key. ChatGPT was helpful and promptly provided me with the code I needed. Although the initial code had some errors and bugs, ChatGPT continued to assist me in resolving them. With the guidance and support of ChatGPT, I successfully debugged the code and ended up with a fully functional integration. The collaboration with ChatGPT proved invaluable in overcoming the challenges and ultimately achieving a working piece of code for the integration.
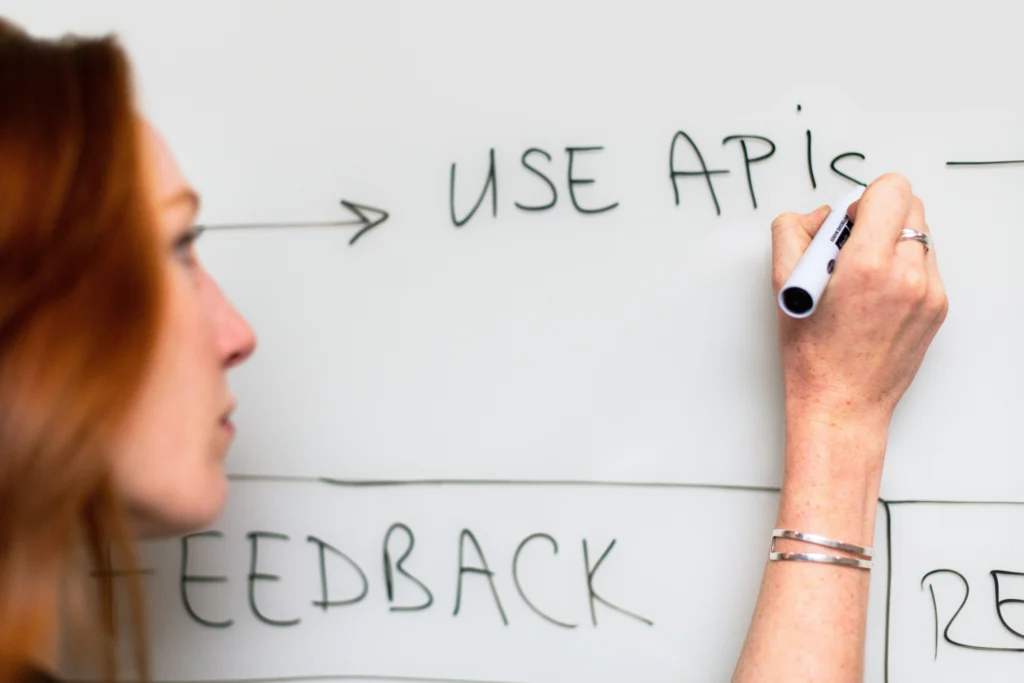
In this blog, we will explore OpenAI API integration into a WordPress website. By leveraging the power of the OpenAI API, we can incorporate natural language processing and generation capabilities into our WordPress site, opening up a wide range of possibilities. We will walk through three key pieces of code that are required for this integration: an HTML code snippet, additional code added to the functions.php file, and a new JavaScript code. Let’s dive into each of these components and understand their role in making the OpenAI integration work seamlessly.
HTML Code
To accompalish OpenAI API integration, the provided HTML code snippet sets up the user interface for our OpenAI integration. It starts with the inclusion of two external JavaScript files. The first script tag imports the Axios library from a CDN (Content Delivery Network). Axios is a popular HTTP client that simplifies making API requests. The second script tag references a custom JavaScript file, openai_custom.js, which contains additional functionality specific to our integration.
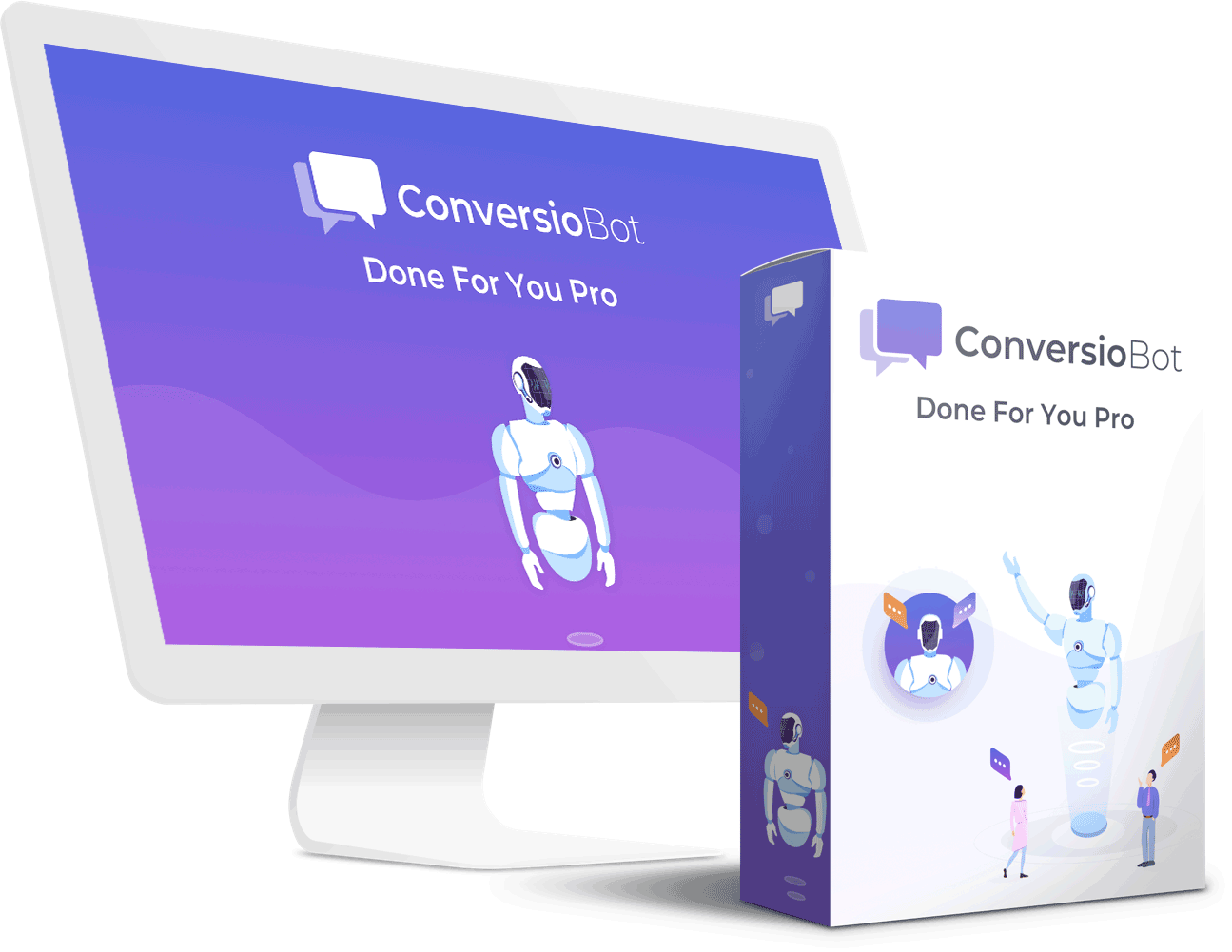
Click to know more about the above product from a Chatbot
Inside the HTML code, we have an <h1>
heading that serves as a title for our integration, labeled “OpenAI Integration.” Below the heading, we create an input field for the user to enter a prompt. This is achieved using the <label>
and <input>
elements, where the input field has an assigned id of “prompt-input” for easy identification.
Next, we have a button with the id “ok-button,” which users will click to trigger the API request. Finally, we create a <div>
element with the id “response-output” to display the response received from the OpenAI API.
<!-- wp:html -->
<title>OpenAI Integration - Testing Playground</title>
<script src="https://cdn.jsdelivr.net/npm/axios/dist/axios.min.js"></script>
<script src="https://techhorizoncity.com/wp-content/themes/consulting/lib/scripts/openai_custom.js"></script>
<h1>OpenAI Integration</h1>
<div>
<label for="prompt-input">Enter a Prompt:</label>
<input type="text" id="prompt-input">
<button id="ok-button">OK</button>
</div>
<div id="response-output"></div>
<script>
function displayResponse(response) {
var responseOutput = document.getElementById('response-output');
responseOutput.innerText = response;
}
</script>
<!-- /wp:html -->
JavaScript Code
The provided JavaScript code defines a function named displayResponse()
, which is responsible for updating the content of the “response-output” div with the response received from the OpenAI API. Inside the function, we use the getElementById()
method to get a reference to the “response-output” div element. By assigning the received response
value to the innerText
property of the div, we update its content with the response text. This function will be used later to display the output of the OpenAI API request to the user.

Explanation of the JavaScript code:
The provided JavaScript code handles the interaction between the user interface and the OpenAI API. It utilizes the Axios library for making HTTP requests and includes an event listener to ensure the code executes after the DOM (Document Object Model) has finished loading.
Upon DOMContentLoaded, the code retrieves references to the input field with the id “prompt-input” and the button with the id “ok-button”. It then performs a check to ensure that both elements are present on the page. If any of them are missing, an error message is logged to the console, and the execution of the code is stopped.
The code adds a click event listener to the “ok-button” element, which triggers the execution of the API request when clicked. Inside the event listener, the necessary variables are set up for the API request:
apiKey
: This variable holds the API key required for authentication. It is set to a specific API key, but you would typically store it securely, such as in an environment variable or a more secure storage location.apiUrl
: This variable specifies the endpoint URL for making the API request. In this case, it is set to the text-davinci-003 engine’s completions endpoint. You can adjust this URL based on the specific OpenAI model and endpoint you want to use.prompt
: This variable captures the value entered by the user in the “prompt-input” field.maxTokens
: This variable determines the maximum number of tokens to generate in the API response.
Next, the code sets up the necessary headers for the API request, including the Content-Type and Authorization. It constructs the data object with the prompt and maxTokens values.
Using the Axios library, the code sends a POST request to the specified API URL, passing the data and headers. It then handles the response using a Promise-based approach. If the request is successful, it extracts the completion text from the response data and calls the displayResponse()
function, passing the completion as an argument. In case of an error, an error message is logged to the console.
The displayResponse()
function, which we discussed earlier, updates the content of the “response-output” div with the received response text.
Overall, this JavaScript code sets up the necessary event listeners, performs the API request, and handles the response to display the output to the user in the designated area on the webpage.
document.addEventListener('DOMContentLoaded', function () {
var promptInput = document.getElementById('prompt-input');
var okButton = document.getElementById('ok-button');
if (!promptInput || !okButton) {
console.error('Required HTML elements not found. Check the IDs of the input field and button.');
return;
}
okButton.addEventListener('click', function () {
var apiKey = 'YOUR API KEY';
var apiUrl = 'https://api.openai.com/v1/engines/text-davinci-003/completions'; // Use the Turbo model endpoint
var prompt = promptInput.value;
var maxTokens = 100;
var headers = {
'Content-Type': 'application/json',
'Authorization': 'Bearer ' + apiKey
};
var data = {
'prompt': prompt,
'max_tokens': maxTokens
};
axios.post(apiUrl, data, { headers })
.then(response => {
var completion = response.data.choices[0].text;
displayResponse(completion); // Call the displayResponse function
})
.catch(error => {
console.error('Error:', error);
});
});
function displayResponse(response) {
var responseOutput = document.getElementById('response-output');
responseOutput.innerText = response;
}
});
Adding Code to Functions.php
Now that we have covered the HTML and JavaScript code, let’s proceed to the next piece of code: adding extra code to the functions.php file.
Explanation of the code addition to functions.php:
The provided code is an addition to the functions.php file in WordPress. It defines a custom function named enqueue_openai_scripts()
and hooks it into the wp_enqueue_scripts
action.
Inside the function, two scripts are enqueued using the wp_enqueue_script()
function:
axios
: This script is loaded from the CDN (Content Delivery Network) provided in the URL'https://cdn.jsdelivr.net/npm/axios/dist/axios.min.js'
. Axios is a popular HTTP client library used for making API requests in JavaScript.openai-custom
: This script is loaded from the specified path within the theme directory using theget_template_directory_uri()
function. It points to the file/lib/scripts/openai_custom.js
, which contains the custom JavaScript code related to the OpenAI integration.
Both scripts are set to load in the footer of the webpage (true
is passed as the last parameter), ensuring that they are loaded after the page content has finished rendering.
By hooking the enqueue_openai_scripts()
function into the wp_enqueue_scripts
action, the scripts will be automatically added to the page whenever the WordPress frontend is generated.
This code addition ensures that the required JavaScript files, axios
and openai-custom
, are properly included in the WordPress website, enabling the functionality for the OpenAI integration to work seamlessly.
function enqueue_openai_scripts() {
wp_enqueue_script('axios', 'https://cdn.jsdelivr.net/npm/axios/dist/axios.min.js', array(), '1.0', true);
wp_enqueue_script( 'openai-custom', get_template_directory_uri() . '/lib/scripts/openai_custom.js', array(), '1.0', true );
}
add_action('wp_enqueue_scripts', 'enqueue_openai_scripts');
Output
After troubleshooting and fine-tuning the code with the help of ChatGPT, I am pleased to present the final output of the OpenAPI integration on my WordPress website. The screen now showcases a user-friendly interface where visitors can enter prompts in the designated input field. Upon clicking the “OK” button, the integration effortlessly communicates with the OpenAI API using the provided API key. The response received from the API is dynamically displayed on the screen within the designated “response-output” area. The seamless integration enhances the user experience by harnessing the power of natural language processing and opens up a world of possibilities on my WordPress website.
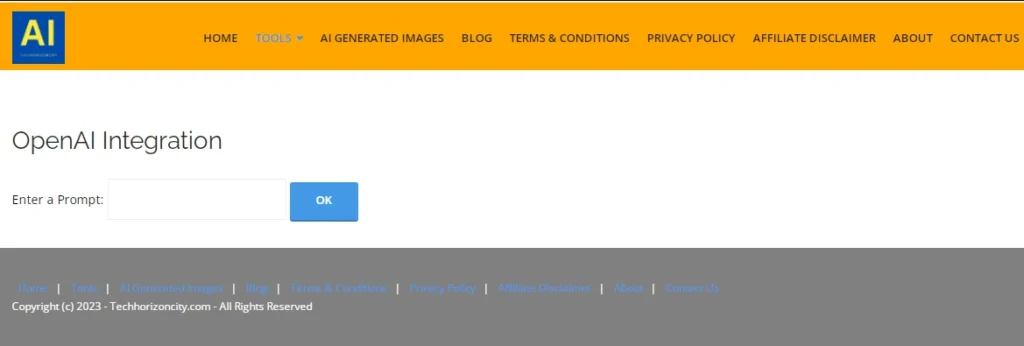

Conclusion
Having successfully implemented this initial piece of code for the OpenAPI integration on my WordPress website, I am now inspired to take it a step further. Recognizing the potential and usefulness of this integration, I plan to leverage this foundation to create powerful tools that cater to the needs of my audience. By expanding on this code, I aim to develop practical applications and functionalities that can provide valuable solutions to users. Whether it’s automating tasks, generating insightful content, or enhancing user interactions, the possibilities are endless. I am excited to embark on this journey of innovation, creating tools that will truly benefit and empower people in their online experiences.
Further Reading: https://techhorizoncity.com/chatgpt-plugins-guide-to-blogging-success/
Link to Email Generator: https://techhorizoncity.com/generate-email/