Table of Contents
Introduction
Finding the perfect gift for someone can often be a challenging task. With countless options available in the market, consumers are often overwhelmed by the choices. In this article, we will explore how to develop a Gift Idea web tool using the OpenAI API, which leverages the power of artificial intelligence to assist consumers in making informed decisions about what to buy based on categories of products, age, and gender. We will also provide the code written in HTML and JavaScript to help you get started. The link to the live project is here: https://activelivingoffers.com/gift-ideas/ and also given at the end of this article.
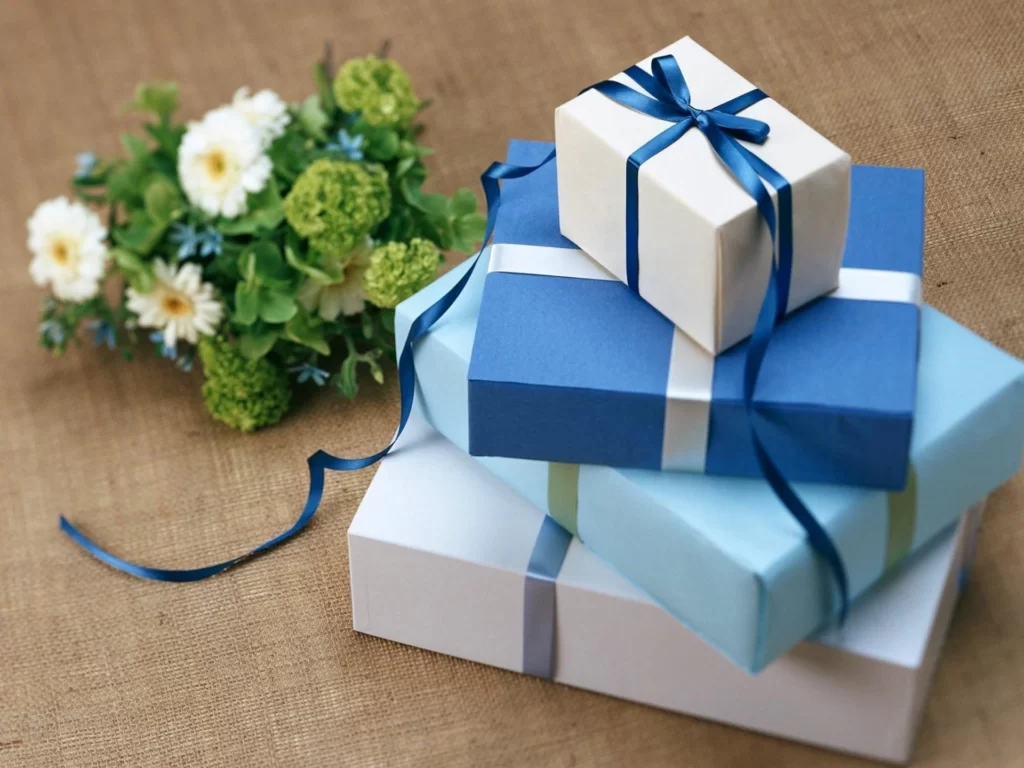
Understanding the Project Scope
Before diving into the development process, it’s essential to define the scope and features of our Gift Idea web tool. The primary goal is to create a user-friendly interface that allows consumers to input their desired category of products, along with the age and gender of the recipient. The tool will then generate a curated list of gift ideas based on these parameters.
Setting up the Development Environment
To begin, make sure you have a basic understanding of HTML, CSS, and JavaScript. These languages will be used to create the user interface and handle the logic of the Gift Idea web tool. Additionally, you’ll need an OpenAI API key to access the GPT-3.5 model for generating gift ideas.
Designing the User Interface
HTML Structure
Create an HTML file and set up the basic structure. Begin with the doctype declaration, followed by the <html>
, <head>
, and <body>
tags. Within the <body>
tag, add elements such as headings, input fields, buttons, and a placeholder to display the generated gift ideas.
<link rel="stylesheet" href="visualcue.css">
<link rel="stylesheet" href="confetti.css">
<form id="myForm">
<fieldset>
<legend>Categories</legend>
<div>
<label><input type="checkbox" name="category" value="Mobiles & Accessories"> Mobiles & Accessories</label>
<label><input type="checkbox" name="category" value="Computers & Accessories"> Computers & Accessories</label>
<label><input type="checkbox" name="category" value="Electronics"> Electronics</label>
<label><input type="checkbox" name="category" value="Men's Fashion"> Men's Fashion</label>
<label><input type="checkbox" name="category" value="Women's Fashion"> Women's Fashion</label></div>
<div>
<label><input type="checkbox" name="category" value="Home & Kitchen"> Home & Kitchen</label>
<label><input type="checkbox" name="category" value="TV, Appliances, & Electronics"> TV, Appliances, & Electronics</label>
<label><input type="checkbox" name="category" value="Health & Personal Care"> Health & Personal Care</label>
<label><input type="checkbox" name="category" value="Beauty"> Beauty</label>
<label><input type="checkbox" name="category" value="Toys & Games"> Toys & Games</label></div>
<div>
<label><input type="checkbox" name="category" value="Books"> Books</label>
<label><input type="checkbox" name="category" value="Sports, Fitness & Outdoors"> Sports, Fitness & Outdoors</label>
<label><input type="checkbox" name="category" value="Baby Products"> Baby Products</label>
<label><input type="checkbox" name="category" value="Automotive"> Automotive</label>
<label><input type="checkbox" name="category" value="Industrial & Scientific"> Industrial & Scientific</label></div></fieldset>
<fieldset>
<legend>Gender</legend> <select name="gender">
<option value="Female">Female</option>
<option value="Male">Male</option>
<option value="Other">Other</option>
</select></fieldset>
<fieldset>
<legend>Age</legend> <select name="age">
<option value="5-10 Years">5-10 Years</option>
<option value="11-15 Years">11-15 Years</option>
<option value="16-20 Years">16-20 Years</option>
<option value="21-25 Years">21-25 Years</option>
<option value="26-30 Years">26-30 Years</option>
<option value="31-35 Years">31-35 Years</option>
<option value="36-40 Years">36-40 Years</option>
<option value="41-45 Years">41-45 Years</option>
<option value="46-50 Years">46-50 Years</option>
<option value="51-55 Years">51-55 Years</option>
<option value="56-60 Years">56-60 Years</option>
<option value="61-65 Years">61-65 Years</option>
<option value="66-70 Years">66-70 Years</option>
<option value="71-75 Years">71-75 Years</option>
<option value="76-80 Years">76-80 Years</option>
<option value="Above 80 Years">Above 80 Years</option>
</select></fieldset>
<button type="button" onclick="submitForm()">OK
</button>
<button type="button" onclick="clearForm()">Clear</button>
</form> <textarea id="output" rows="10" cols="50" readonly=""></textarea>
<h4>Amazon Link:</h4>
<div id="amazonLink"></div>
<div class="confetti-container"></div>
<div id="loading-spinner" class="spinner"></div>
<script src="https://cdn.jsdelivr.net/npm/axios/dist/axios.min.js"></script>
<script src="getgiftideas.js"></script>
CSS Styling
Enhance the appearance of your web tool using CSS. Apply appropriate styles to the elements, such as font size, colors, spacing, and layout, to create an intuitive and visually appealing user interface.
Handling User Input
Use JavaScript to capture the user’s input from the form fields. Write a JavaScript function that triggers when the user clicks the submit button. Within this function, extract the category, age, and gender values entered by the user.
Communicating with OpenAI API
OpenAI API Setup
Integrate the OpenAI API by making an HTTP request to their API endpoint. You’ll need to include your API key in the request headers. Ensure you have the necessary libraries and dependencies installed.
Sending Requests
Using the extracted user input, formulate a request to the OpenAI API, specifying the appropriate parameters. Construct a JSON object containing the category, age, and gender values, and pass it as the payload in the API request.
Receiving and Processing Responses
Upon receiving a response from the OpenAI API, extract the gift ideas generated by the GPT-3.5 model. You can parse the response JSON and access the relevant information. Organize the ideas into a presentable format, such as a list or grid.
Displaying Gift Ideas
HTML Manipulation
Using JavaScript, manipulate the HTML elements of your web tool to display the generated gift ideas. You can dynamically create new elements, update existing elements, or modify the CSS classes of elements to accommodate the generated content.
Enhancing User Experience
To make your Gift Idea web tool more user-friendly, consider implementing additional features such as filtering options, sorting mechanisms, or the ability to save or share the gift ideas. These features can improve usability and provide a more personalized experience to the users.
Generating Amazon Links for Easy Shopping
In addition to providing curated gift ideas, let’s enhance our Gift Idea web tool further by generating Amazon links based on the user’s input. This feature will allow users to conveniently navigate to the Amazon website and explore or purchase products related to their search.
Retrieving User Input
Modify the JavaScript function responsible for capturing user input to store the category, age, and gender values in variables. You can use these variables to construct the Amazon search query later on.
function submitForm() {
displayVisualCue();
var categories = [];
var categoryCheckboxes = document.getElementsByName('category');
for (var i = 0; i < categoryCheckboxes.length; i++) {
if (categoryCheckboxes[i].checked) {
categories.push(categoryCheckboxes[i].value);
}
}
var gender = document.getElementsByName('gender')[0].value;
var age = document.getElementsByName('age')[0].value;
var requestData = {
prompt: 'Please suggest gift ideas for the following from Amazon.in. Do not give any URLs: Categories: ' + categories.join(', ') + '\nGender: ' + gender + '\nAge: ' + age,
max_tokens: 1000,
n: 1
};
var config = {
headers: {
'Content-Type': 'application/json',
'Authorization': 'YOUR API KEY'
}
};
axios.post('https://api.openai.com/v1/engines/text-davinci-003/completions', requestData, config)
.then(function (response) {
var output = response.data.choices[0].text.trim();
var affiliateLink = generateAffiliateLink(output, categories);
var outputWithLink = 'Click the link at the bottom of the screen to start shopping: ' + '' + '\n\n' + output;
document.getElementById('output').value = outputWithLink;
hideVisualCue();
createConfetti();
})
.catch(function (error) {
alert('An error occurred while calling the API.');
console.error(error);
});
}
function clearForm() {
var categoryCheckboxes = document.getElementsByName('category');
for (var i = 0; i < categoryCheckboxes.length; i++) {
categoryCheckboxes[i].checked = false;
}
document.getElementsByName('gender')[0].value = 'Male';
document.getElementsByName('age')[0].value = '5-10 Years';
document.getElementById('output').value = '';
document.getElementById('amazonLink').innerHTML = '';
}
function generateAffiliateLink(text, categories) {
var affiliateStoreId = 'YOUR AMAZON STORE ID IF YOU ARE AN AFFILIATE';
var baseUrl = 'https://www.amazon.in';
var keywordParam = categories.map(function(category) {
return 'k=' + encodeURIComponent(category);
}).join('&');
var trackingParams = 'linkCode=ll2&language=en_IN&ref_=as_li_ss_tl';
var affiliateLink = `${baseUrl}/s?${keywordParam}&tag=${affiliateStoreId}&${trackingParams}`;
var outputWithLink = 'Click on this link to buy: <a href="' + affiliateLink + '" target="_blank">' + affiliateLink + '</a>';
document.getElementById('amazonLink').innerHTML = outputWithLink;
return affiliateLink;
}
// Declare the visualcue function
function displayVisualCue() {
var spinner = document.getElementById("loading-spinner");
spinner.style.display = "block";
}
function hideVisualCue() {
var spinner = document.getElementById("loading-spinner");
spinner.style.display = "none";
}
let confettiRunning = true;
function createConfetti() {
if (!confettiRunning) return; // Stop creating confetti if the flag is false
const colors = ['#f55', '#0af', '#ff0', '#0f0', '#f0f', '#00f', '#f80']; // List of colors
const duration = Math.floor(Math.random() * 4000) + 8000; // Random duration between 5 and 8 seconds
const container = document.querySelector('.confetti-container');
const viewportHeight = window.innerHeight; // Get the viewport height
const adjustmentValue = 500; // Number of pixels to adjust the starting position
const confettiStartPosition = Math.max(document.documentElement.scrollTop, window.pageYOffset) + viewportHeight - adjustmentValue; // Adjust the starting position of the confetti
for (let i = 0; i < 50; i++) {
const confetti = document.createElement('div');
confetti.classList.add('confetti');
confetti.style.left = Math.random() * 100 + '%';
confetti.style.animationDelay = Math.random() * 3 + 's';
confetti.style.backgroundColor = colors[Math.floor(Math.random() * colors.length)]; // Apply random color
confetti.style.top = confettiStartPosition + 'px'; // Set the adjusted starting position
container.appendChild(confetti);
}
setTimeout(() => {
const confettiElements = document.querySelectorAll('.confetti');
confettiElements.forEach((confetti) => {
confetti.remove();
});
confettiRunning = false; // Set the flag to false after the confetti is removed
}, 5000); // Change the duration to 5000 milliseconds (5 seconds)
}
Building the Amazon Link
Using the retrieved user input, create a function that constructs the Amazon link. Start by forming the base URL for Amazon’s search page. Append the relevant search parameters to the URL based on the category, age, and gender values provided by the user.
Displaying the Amazon Link
Using JavaScript, dynamically generate an anchor tag (<a>
) element within your HTML code. Set the href
attribute of the anchor tag to the constructed Amazon link. Additionally, provide a visually appealing representation of the link, such as a button or clickable text, for users to click on.
Conclusion
By adding the ability to generate Amazon links based on user input, our Gift Idea web tool becomes an even more valuable resource for consumers. With a single click, users can navigate to the Amazon website and conveniently explore a wide range of products aligned with their desired category, age, and gender. This integration provides a seamless shopping experience and enhances the overall usability and usefulness of the tool.
Remember to comply with Amazon’s guidelines and policies regarding affiliate links and usage restrictions. Make sure to familiarize yourself with their terms of service and any specific requirements for displaying Amazon links.
With the combination of curated gift ideas and direct access to Amazon’s vast product selection, your web tool is poised to become a go-to resource for individuals seeking thoughtful and personalized gift suggestions. Happy coding and may your Gift Idea web tool bring joy and convenience to users in their gift-giving endeavors!
Project Link: https://activelivingoffers.com/gift-ideas/
Further Reading: https://techhorizoncity.com/optimize-youtube-using-simple-free-tool/