Table of Contents
Introduction
As crypto market continues to evolve, the demand for accurate and timely price information becomes crucial. In this tutorial/article/blog, we’ll examine how to build a website that predicts bitcoin values using JavaScript and the Binance API. While this example focuses on retrieving real-time price data, it serves as a starting point to demonstrate the potential for obtaining more data and creating a comprehensive crypto prediction website.
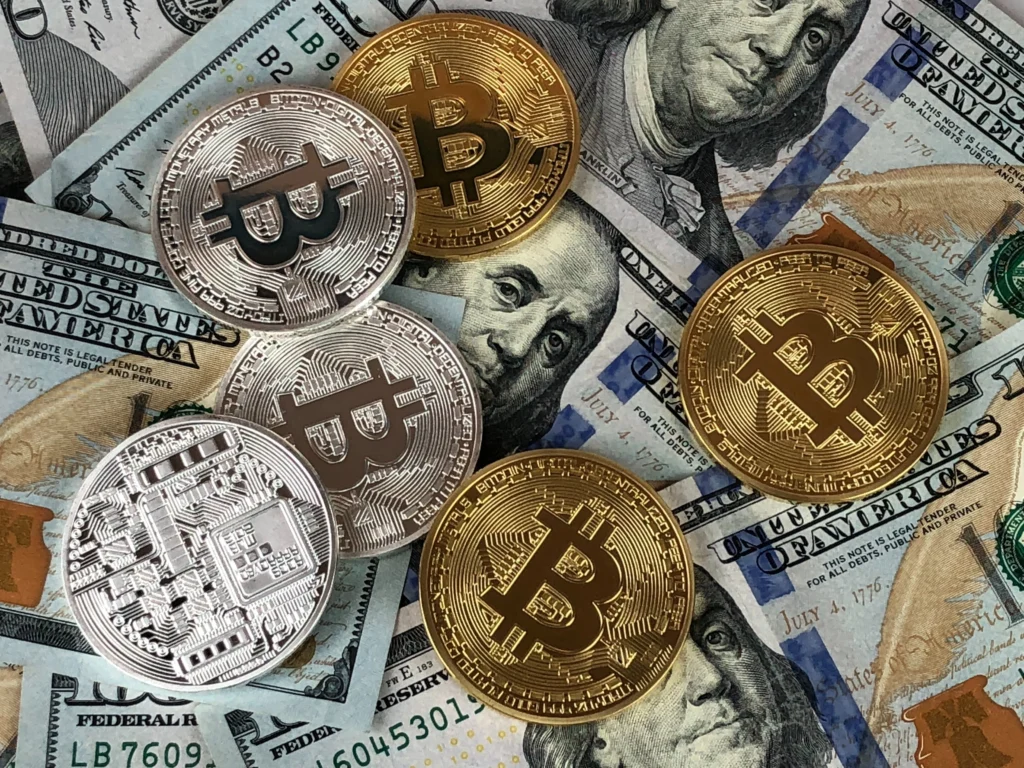
Understanding the Binance API
Binance, a leading cryptocurrency exchange, provides a powerful API that allows developers to interact with their platform programmatically. Through this API, developers gain access to various endpoints for retrieving market data, historical prices, and real-time ticker information.
Setting Up the Development Environment
Begin by setting up your development environment. Please ensure your computer has a text editor and a web browser installed. Open the project folder you just created in your favourite text editor.
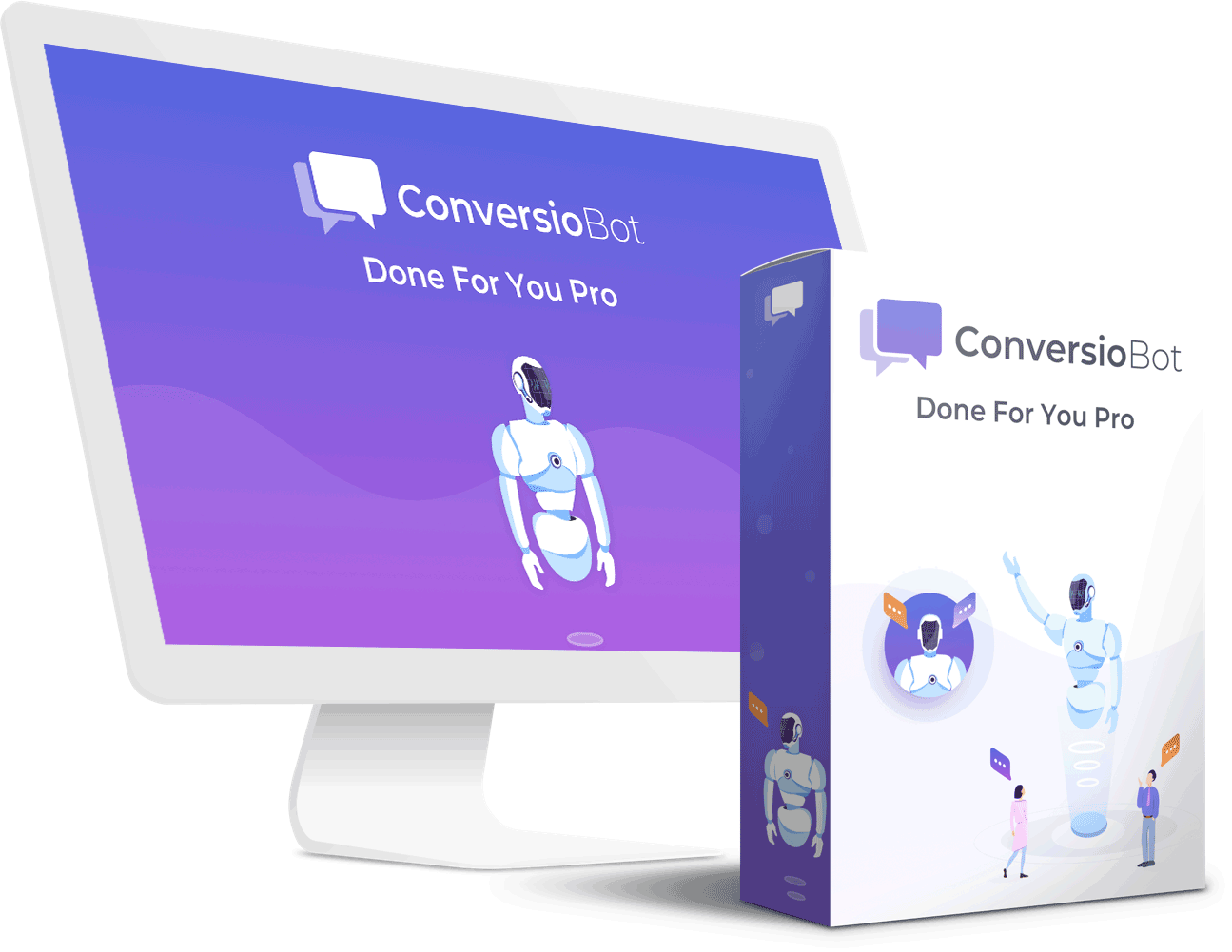
Click to know more about the above product from a Chatbot
HTML Structure
Create the basic HTML structure for your web-page. Include the necessary HTML tags such as <html>
, <head>
, and <body>
. Set up a drop-down menu using the <select>
element, which users can utilize to select the cryptocurrency symbol. The drop-down will be populated dynamically later using the Binance API.
<title>Crypto Symbols</title>
<style>
.output {<br />
margin-top: 10px;<br />
padding: 5px;<br />
border: 1px solid #ccc;<br />
width: 300px;<br />
}<br />
</style> <script src="crypto_price.js"></script>
<script src="get_all_crypto_symbols.js"></script>
<h1>Crypto Price</h1>
<label for="symbol">Select a symbol:</label>
<select id="symbol"></select>
<button onclick="getData()">OK</button>
<div class="output-response">
<label for="price">Price in USD:</label>
<input type="text" id="price" readonly=""></div>
<script><br />
// Function to retrieve all symbols and generate dropdown options<br />
function getAllSymbols() {<br />
var API_ENDPOINT = 'https://api.binance.com/api/v3/exchangeInfo';</p>
<p> fetch(API_ENDPOINT)<br />
.then(response => response.json())<br />
.then(data => {<br />
if (data && data.symbols) {<br />
var symbols = data.symbols.map(symbol => symbol.symbol);<br />
generateDropdownOptions(symbols);<br />
} else {<br />
console.log('Failed to retrieve symbols');<br />
}<br />
})<br />
.catch(error => console.error(error));<br />
}</p>
<p> // Function to generate dropdown options<br />
function generateDropdownOptions(symbols) {<br />
var symbolSelect = document.getElementById('symbol');</p>
<p> // Clear any existing options<br />
symbolSelect.innerHTML = '';</p>
<p> // Generate options for each symbol<br />
symbols.forEach(symbol => {<br />
var option = document.createElement('option');<br />
option.value = symbol;<br />
option.textContent = symbol;<br />
symbolSelect.appendChild(option);<br />
});<br />
}</p>
<p> // Call getAllSymbols() when the page loads to populate the dropdown<br />
document.addEventListener('DOMContentLoaded', getAllSymbols);</p>
<p> // Function to get data based on selected symbol<br />
function getData() {<br />
var symbolSelect = document.getElementById('symbol');<br />
var selectedSymbol = symbolSelect.value;</p>
<p> var API_ENDPOINT = 'https://api.binance.com/api/v3/ticker/price';</p>
<p> var url = new URL(API_ENDPOINT);<br />
url.searchParams.append('symbol', selectedSymbol);</p>
<p> fetch(url)<br />
.then(response => response.json())<br />
.then(data => {<br />
if (data) {<br />
var price = parseFloat(data.price);<br />
console.log(`Current ${selectedSymbol} price: ${price}`);<br />
displayPrice(price);<br />
} else {<br />
console.log(`Failed to get price for symbol ${selectedSymbol}`);<br />
}<br />
})<br />
.catch(error => console.error(error));<br />
}</p>
<p> // Function to display the price<br />
function displayPrice(price) {<br />
var priceInput = document.getElementById('price');<br />
priceInput.value = price;<br />
}<br />
</script>
Fetching Crypto Symbols
To populate the drop-down with cryptocurrency symbols, make an API call to Binance using JavaScript’s fetch()
function. Send a GET request to the Binance API endpoint that provides a list of available symbols. Parse the response and extract the symbol names. Then, dynamically create <option>
elements and append them to the drop-down menu.
Retrieving Real-Time Price Data
Make another API call after choosing a symbol from the drop-down menu to get the symbol’s current price information. Attach an event listener to the drop-down, listening for the ‘change’ event. When the selection changes, extract the chosen symbol and send an API request to Binance to obtain the current price for that symbol. Display the price on the web-page using an HTML element.
Javascript (get_all_crypto_symbols.js)
Javascript for get_all_crypto_symbols.js
function getAllSymbols() {
var API_ENDPOINT = 'https://api.binance.com/api/v3/exchangeInfo';
fetch(API_ENDPOINT)
.then(response => response.json())
.then(data => {
if (data && data.symbols) {
var symbols = data.symbols.map(symbol => symbol.symbol);
generateDropdownOptions(symbols);
} else {
console.log('Failed to retrieve symbols');
}
})
.catch(error => console.error(error));
}
function generateDropdownOptions(symbols) {
var symbolSelect = document.getElementById('symbol');
// Clear any existing options
symbolSelect.innerHTML = '';
// Generate options for each symbol
symbols.forEach(symbol => {
var option = document.createElement('option');
option.value = symbol;
option.textContent = symbol;
symbolSelect.appendChild(option);
});
}
// Call getAllSymbols() when the page loads to populate the dropdown
document.addEventListener('DOMContentLoaded', getAllSymbols);
Javascript for Crypto_price.js
function getData() {
var symbolSelect = document.getElementById('symbol');
var selectedSymbol = symbolSelect.value;
var API_ENDPOINT = 'https://api.binance.com/api/v3/ticker/price';
var url = new URL(API_ENDPOINT);
url.searchParams.append('symbol', selectedSymbol);
fetch(url)
.then(response => response.json())
.then(data => {
if (data) {
var price = parseFloat(data.price);
console.log(`Current ${selectedSymbol} price: ${price}`);
displayPrice(price);
} else {
console.log(`Failed to get price for symbol ${selectedSymbol}`);
}
})
.catch(error => console.error(error));
}
function displayPrice(price) {
var priceInput = document.getElementById('price');
priceInput.value = price;
}
Expanding Data Sources
While this example focuses on retrieving real-time price data, it’s important to highlight that you can extend your website’s functionality by incorporating additional data sources. For instance, you can explore integrating historical price data, market sentiment analysis, social media data, or even news sentiment indicators. These additional data points can enhance the accuracy and reliability of your prediction model.
Building Advanced Prediction Models
Building Advanced Prediction Models: To enhance your crypto prediction website, you can implement more sophisticated prediction models. While this example demonstrates a simple implementation, consider exploring techniques such as moving averages, exponential smoothing, or even machine learning algorithms. These advanced models can analyze historical price patterns, market trends, and other relevant factors to provide more accurate predictions.

Enhancing User Interface and Visualization
By adding visually appealing CSS styles to your website, you may enhance the user experience. Utilize CSS frameworks like Bootstrap or create custom styles tailored to your design preferences. Consider incorporating interactive charts or graphs to visualize historical price data, prediction trends, and other relevant information. These visual elements provide users with a comprehensive understanding of the crypto market dynamics.
Conclusion
In this blog, we explored the initial steps to build a cryptocurrency price prediction website using JavaScript and the Binance API. While the example focused on retrieving real-time price data, it showcased the potential to expand and integrate additional data sources to enhance prediction accuracy. By incorporating advanced prediction models and visualizing data effectively, you can create a robust and informative crypto prediction website. Remember to leverage the vast resources available and continuously refine your models to stay ahead in the ever-evolving crypto market.
Further Reading: https://techhorizoncity.com/building-a-profitable-tools-website/